Module Federation on Micro Frontend Architecture
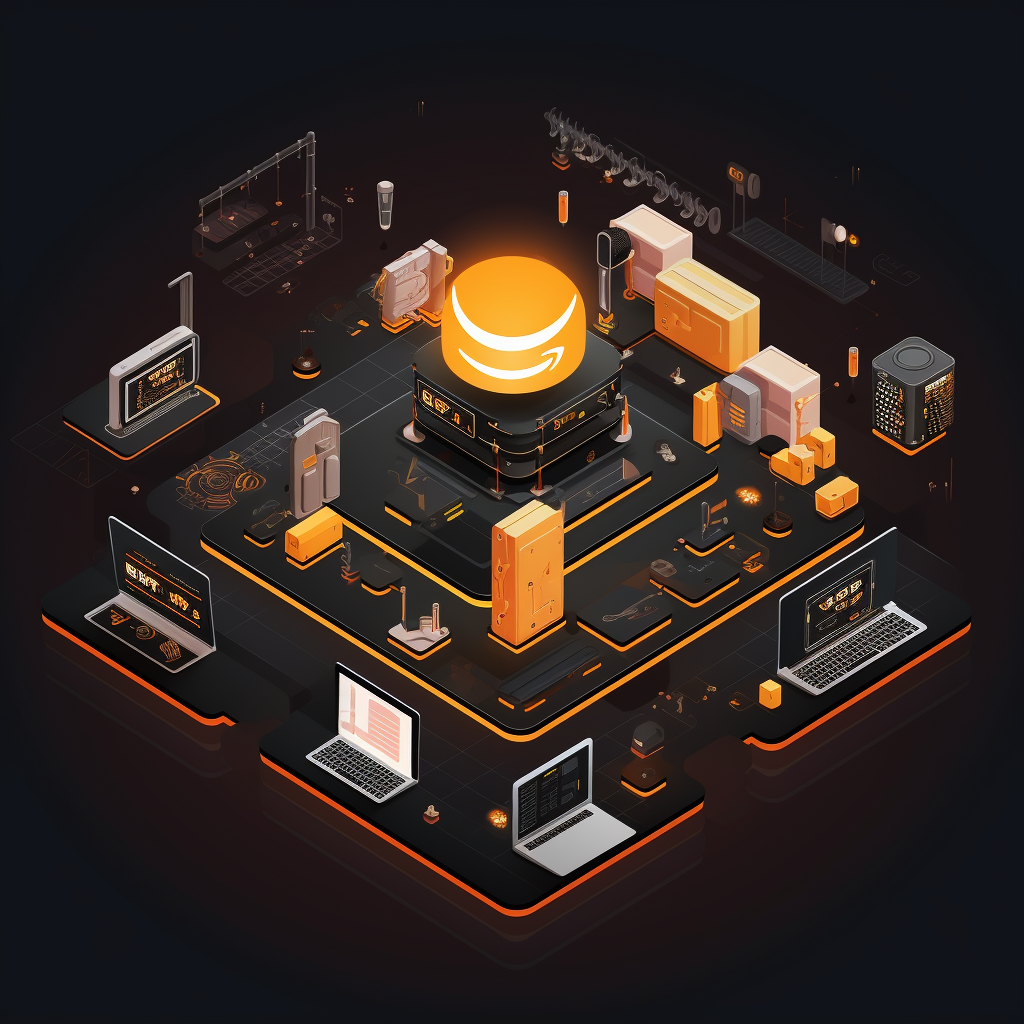
Module Federation is a powerful feature that enables the development of micro-frontends by allowing different frontend applications to share code and resources at runtime. This example will demonstrate how to set up Module Federation in a micro-frontend architecture using Webpack 5, which is the underlying technology that powers Module Federation.
Step 1: Setup Host Application
First, let's set up the host application. The host is the main application that will consume components or modules from other applications (remotes).
Load the Remote Module:In your host application, you can now load and use the remote module. For example, if the remote application exposes a component named RemoteComponent
, you can import it like this:
import React from 'react';
import ReactDOM from 'react-dom';
import RemoteComponent from 'remoteApp/RemoteComponent';
function App() {
return (
<div>
<h1>Host Application</h1>
<RemoteComponent />
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
Configure Webpack:Create a webpack.config.js
file in the root of your project. This configuration will include the ModuleFederationPlugin
to expose or consume modules.
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin');
module.exports = {
mode: 'development',
devServer: {
contentBase: './dist',
port: 3000,
},
plugins: [
new ModuleFederationPlugin({
name: 'host',
remotes: {
// This is where we'll specify the remote(s) we want to consume
remoteApp: 'remoteApp@http://localhost:3001/remoteEntry.js',
},
}),
],
};
In this configuration, remoteApp
is the name we've given to the remote application, and http://localhost:3001/remoteEntry.js
is the URL where the remote's remoteEntry.js
file can be found.
Create a New Webpack Project:If you're starting from scratch, create a new directory for your host application and initialize it with a package.json file. Then, install Webpack and its CLI:
npm init -y
npm install webpack webpack-cli --save-dev
Step 2: Setup Remote Application
The remote application is the one sharing its code with the host.
Run Both Applications:Start both applications. The host should be able to load and display the RemoteComponent
from the remote application.
# In the host directory
npx webpack serve
# In the remote directory
npx webpack serve
Create the Shared Component:In the remote application, create a RemoteComponent.js
file under src
:
import React from 'react';
const RemoteComponent = () => {
return <div>This is a Remote Component</div>;
};
export default RemoteComponent;
Configure Webpack:Create a webpack.config.js
file in the root of your remote application. This configuration will expose a module to be consumed by the host.
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin');
module.exports = {
mode: 'development',
devServer: {
contentBase: './dist',
port: 3001,
},
plugins: [
new ModuleFederationPlugin({
name: 'remoteApp',
filename: 'remoteEntry.js',
exposes: {
'./RemoteComponent': './src/RemoteComponent',
},
}),
],
};
Here, remoteApp
is the name of the remote application, remoteEntry.js
is the file that will be generated and contains the information about the exposed modules, and ./RemoteComponent
is the path to the component we want to share.
Create a New Webpack Project: Similar to the host, create a new directory for the remote application and initialize it with a package.json file. Install Webpack and its CLI:
npm init -y
npm install webpack webpack-cli --save-dev
This example demonstrates the basic setup for using Module Federation in a micro-frontend architecture. It allows for the sharing of components or modules between different applications at runtime, enabling a more modular and scalable frontend development process.